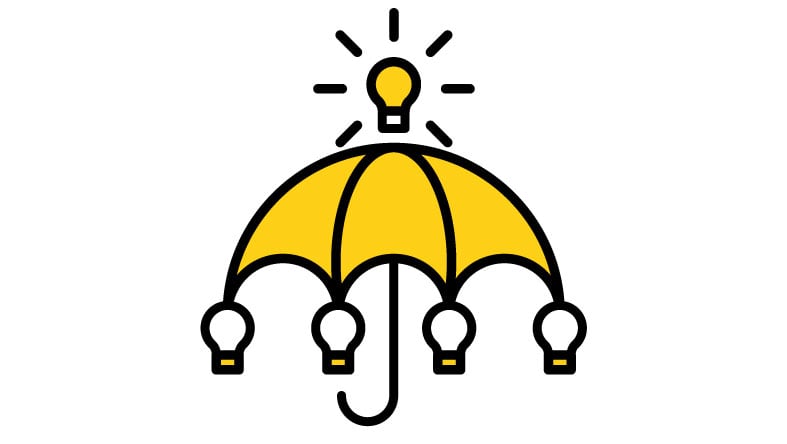
The Power of Concepts
Type Constraints in C++ Templates: The Power of Concepts
C++ is a programming language known for its performance and flexibility, largely driven by its templates. However, the flexibility templates offer sometimes comes at the cost of developer frustration. Without clear ways to constrain types, templates could lead to cryptic error messages and maintenance headaches. Enter Concepts, introduced with C++20, as a solution to simplify type constraints and improve readability. This article will dive into Concepts, starting with the old methods and exploring their modern applications.
Before Concepts: The Old Ways
Before Concepts, C++ developers relied on various techniques to enforce type constraints in templates. While functional, these methods often resulted in verbose, complex, and error-prone code. Let’s explore these approaches and their drawbacks.
1. std::enable_if
and SFINAE
Introduced with C++11, std::enable_if
was the go-to tool for enforcing type constraints. This mechanism used SFINAE (Substitution Failure Is Not An Error), where a template would be ignored if its type constraints were not met.
Example: Using std::enable_if
#include <type_traits>
#include <iostream>
// Works only for integral types
template <typename T>
typename std::enable_if<std::is_integral<T>::value, void>::type
print(T value) {
std::cout << "Integer: " << value << std::endl;
}
// Works only for floating-point types
template <typename T>
typename std::enable_if<std::is_floating_point<T>::value, void>::type
print(T value) {
std::cout << "Floating-point: " << value << std::endl;
}
int main() {
print(10); // Output: Integer: 10
print(3.14); // Output: Floating-point: 3.14
// print("Text"); // Compilation error
return 0;
}
While std::enable_if
worked, it came with issues:
- Complex Error Messages: Incompatible types often resulted in verbose and cryptic compiler errors.
- Reduced Readability: The syntax was hard to follow, especially with nested conditions.
2. static_assert
for Type Validation
Another approach was using static_assert
, introduced in C++11. It enforced constraints at compile time by ensuring that a type met a specific condition.
Example: Using static_assert
#include <type_traits>
#include <iostream>
template <typename T>
void print(T value) {
static_assert(std::is_integral<T>::value, "T must be an integral type");
std::cout << "Value: " << value << std::endl;
}
int main() {
print(10); // Output: Value: 10
// print(3.14); // Compilation error: T must be an integral type
return 0;
}
While this method improved clarity, it was still limited:
- No Overload Selection: Static assertions did not allow for clean handling of multiple type-specific implementations.
- Repetitive Code: Constraints needed to be written multiple times across different functions.
3. Tag Dispatching
Developers often used Tag Dispatching to specialize template behavior based on type traits. This technique relied on helper types like std::true_type
and std::false_type
.
Example: Tag Dispatching
#include <type_traits>
#include <iostream>
// Integer-specific implementation
void process_impl(int, std::true_type) {
std::cout << "Integer type" << std::endl;
}
// Floating-point-specific implementation
void process_impl(double, std::false_type) {
std::cout << "Floating-point type" << std::endl;
}
// Generic function selecting implementation based on type
template <typename T>
void process(T value) {
process_impl(value, std::is_integral<T>{});
}
int main() {
process(10); // Output: Integer type
process(3.14); // Output: Floating-point type
return 0;
}
While powerful, Tag Dispatching made the codebase harder to understand and maintain.
4. Function Overloading
The simplest solution was to use function overloading to provide separate implementations for different types.
Example: Function Overloading
#include <iostream>
// For integers
void print(int value) {
std::cout << "Integer: " << value << std::endl;
}
// For floating-point numbers
void print(double value) {
std::cout << "Floating-point: " << value << std::endl;
}
int main() {
print(10); // Output: Integer: 10
print(3.14); // Output: Floating-point: 3.14
return 0;
}
While straightforward, this approach lacked flexibility for generalized constraints in templates.
Concepts: A Modern Solution
C++20 introduced Concepts, revolutionizing template type constraints. Concepts allow developers to express type requirements in a clean, concise, and reusable manner. They make templates easier to understand and produce more meaningful error messages.
Using Concepts
Concepts are defined with the concept
keyword and return a boolean value based on type conditions. They can be applied to template parameters to enforce constraints.
Example: A Simple Concept
#include <concepts>
#include <iostream>
// Concept to check if a type is integral
template <typename T>
concept IsIntegral = std::is_integral_v<T>;
void print(IsIntegral auto value) {
std::cout << "Value: " << value << std::endl;
}
int main() {
print(10); // Output: Value: 10
// print(3.14); // Compilation error
return 0;
}
Varieties of Concepts Usage
Concepts can be applied in various ways, offering flexibility in template programming:
-
Constrained Templates
template <IsIntegral T> void print(T value) { std::cout << "Value: " << value << std::endl; }
-
Constrained Function Parameters
void print(IsIntegral auto value) { std::cout << "Value: " << value << std::endl; }
-
Nested Concepts
template <typename T> concept Addable = requires(T a, T b) { { a + b } -> std::convertible_to<T>; }; void add(Addable auto a, Addable auto b) { std::cout << "Sum: " << (a + b) << std::endl; }
-
Combining Concepts
template <typename T> concept IntegralOrFloatingPoint = std::is_integral_v<T> || std::is_floating_point_v<T>; void process(IntegralOrFloatingPoint auto value) { std::cout << "Processing: " << value << std::endl; }
Conclusion
Concepts mark a significant leap in the evolution of C++. Compared to the older methods, they offer:
- Cleaner Syntax: Constraints are easy to write and understand.
- Reusability: Concepts can be defined once and reused across multiple templates.
- Meaningful Error Messages: Developers spend less time deciphering cryptic compiler errors.
By adopting Concepts in your modern C++ projects, you not only write more expressive and maintainable code but also embrace the future of type-safe template programming. Why not give them a try and see the difference for yourself?